Презентация - "Презентация по Программированию на тему "Python Tuples" в рамках Исследования в действии"
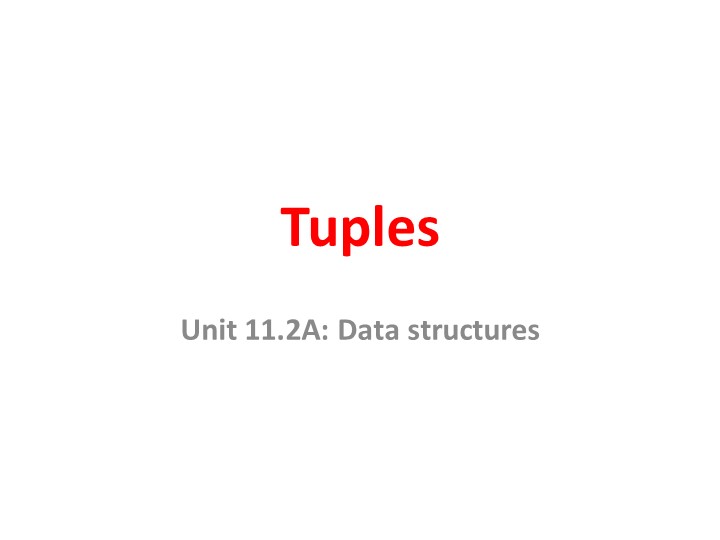
- Презентации / Другие презентации
- 0
- 21.10.23
Просмотреть и скачать презентацию на тему "Презентация по Программированию на тему "Python Tuples" в рамках Исследования в действии"
Lesson objectives
11.2.4.1 create a tuple;
11.2.2.1 perform access to the elements of strings, lists, tuples;
11.2.4.2 convert from one data structure to another;
11.4.3.2 solve applied problems of various subject areas.
https://holypython.com/beginner-python-exercises/exercise-7-python-tuples/
Python Tuple Exercises
Task
Create a Num Tuple
Create a mixed Tuple
Find the maximum, minimum and sum of Num tuple
Zipped two Tuples
Add “5” element to the Num Tuple and Output it.
[5]
2. Python program to remove all tuples of length K
Input: [(1, 4), (2), (4,5,6,8), (26), (3, 0, 1), (4)]
Output: [(1, 4), (4, 5, 6, 8), (3, 0, 1)]
k = 1
Python program to remove all tuples of length K
Write a
Input: [(1, 4), (2), (4,5,6,8), (26), (3, 0, 1), (4)]
Output: [(1, 4), (4, 5, 6, 8), (3, 0, 1)]
k = 1
Tuples
A tuple is a collection of objects which is ordered and immutable. Tuples are similar to lists, the main difference list the immutability.
In Python tuples are written with round brackets and comma separated values.
Tuples
A tuple is a collection of objects which is ordered and immutable. Tuples are similar to lists, the main difference list the immutability.
In Python tuples are written with round brackets and comma separated values.
my_tuple = ("Max", 28, "New York")
Reasons to use a tuple over a list
Generally used for objects that belong together.
Use tuple for heterogeneous (different) data types and list for homogeneous (similar) data types.
Since tuple are immutable, iterating through tuple is slightly faster than with list.
Tuples with their immutable elements can be used as key for a dictionary.
This is not possible with lists.
If you have data that doesn't change, implementing it as tuple will guarantee that it remains write-protected.
Create a tuple
tuple_1 = ("Max", 28, "New York")
tuple_2 = "Linda", 25, "Miami"
tuple_3=(25,)
print(tuple_1)
print(tuple_2)
print(tuple_3)
tuple_4 = tuple([1,2,3])
print(tuple_4)
Access elements
tuple_1 = ("Max", 28, "New York")
item = tuple_1[0]
print(item)
item = tuple_1[-1]
print(item)
item = tuple_1[2]
print(item)
Add or change items
tuple_1 = ("Max", 28, "New York")
tuple_1[2] = "Boston“
print(tuple_1)
Not possible and will raise a TypeError.
Check if an item exists
tuple_1 = ("Max", 28, "New York")
if "New York" in tuple_1:
print("yes")
else:
print("no")
Useful methods
my_tuple = ('a','p','p','l','e',)
# len() : get the number of elements in a tuple
print(len(my_tuple))
# count(x) : Return the number of items that is equal to x
print(my_tuple.count('p'))
# index(x) : Return index of first item that is equal to x
print(my_tuple.index('l'))
# repetition
my_tuple = ('a', 'b') * 5
print(my_tuple)
Useful methods
# concatenation
my_tuple = (1,2,3) + (4,5,6)
print(my_tuple)
# convert list to a tuple and vice versa
my_list = ['a', 'b', 'c', 'd']
list_to_tuple = tuple(my_list)
print(list_to_tuple)
tuple_to_list = list(list_to_tuple)
print(tuple_to_list)
# convert string to tuple
string_to_tuple = tuple('Hello')
print(string_to_tuple)